
Return multiple arguments via return in functions
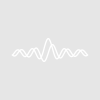
ankit7540
Thu, 05/02/2024 - 05:27 am
Hello all,
I am using function to fit a peak in a 1D wave and get the fit coefficients. It works very well, however, I wonder if I could return all the fit coefficients using the return statement.
/////////////////////////////////////////////////////////////////////////////////////////////////////////////
function analyze_peak(input, xaxis, startP, endP)
wave input
wave xaxis
variable startP
variable endP
// get initial guess
variable baseline = ( input[0] + input[1] ) /2
wavestats /Q input
variable guess_ampl = abs(V_max - V_min)
variable nRows = dimsize( input, 0)
make /o /d /n=(5) init_guess // gaussian fit coefs
// --- assign initial guess ----
init_guess [0] = baseline
init_guess [1] = 1.7e-8
init_guess [2] = guess_ampl
init_guess [3] = 1748
init_guess [4] = 14
// -----------------------------------
//print "initial : ", init_guess
FuncFit /Q gauss_slope init_guess input /X=xaxis
// test fit
make /o /d /n=(nRows) residual = input - ( gauss_slope( init_guess, xaxis ) )
make /o /d /n=(nRows) resd_abs = abs(residual)
// statistics on residual
wavestats /Q residual
variable maxVal = wavemax(resd_abs)
//print maxVal, v_avg, v_sdev
if ( ( maxVal < 3.6e-4 ) && (v_sdev < 5e-5) )
//print "optimized : ", init_guess
return init_guess[2] // amplitude (passing this to other function)
else
return 0 // amplitude
endif
killwaves /Z init_guess, residual, resd_abs
end
/////////////////////////////////////////////////////////////////////////////////////////////////////////////
function analyze_peak(input, xaxis, startP, endP)
wave input
wave xaxis
variable startP
variable endP
// get initial guess
variable baseline = ( input[0] + input[1] ) /2
wavestats /Q input
variable guess_ampl = abs(V_max - V_min)
variable nRows = dimsize( input, 0)
make /o /d /n=(5) init_guess // gaussian fit coefs
// --- assign initial guess ----
init_guess [0] = baseline
init_guess [1] = 1.7e-8
init_guess [2] = guess_ampl
init_guess [3] = 1748
init_guess [4] = 14
// -----------------------------------
//print "initial : ", init_guess
FuncFit /Q gauss_slope init_guess input /X=xaxis
// test fit
make /o /d /n=(nRows) residual = input - ( gauss_slope( init_guess, xaxis ) )
make /o /d /n=(nRows) resd_abs = abs(residual)
// statistics on residual
wavestats /Q residual
variable maxVal = wavemax(resd_abs)
//print maxVal, v_avg, v_sdev
if ( ( maxVal < 3.6e-4 ) && (v_sdev < 5e-5) )
//print "optimized : ", init_guess
return init_guess[2] // amplitude (passing this to other function)
else
return 0 // amplitude
endif
killwaves /Z init_guess, residual, resd_abs
end
/////////////////////////////////////////////////////////////////////////////////////////////////////////////
One way is to use a temporary 1D wave to keep the fit coefficients and use it to move the fit coeffcients to other functions / programs. Is there better way to do that.
Thanks.
Using a 1D wave is probably the easiest, and certainly the most frequently used way.
You can return multiple parameters, execute DisplayHelpTopic "Multiple Return Syntax" for details.
Since waves are passed by reference, you can pass a wave to a function and reset the values within the function.
Or you can write a function that returns a wave. That way you would create the coefficient wave in the function and return the wave for use elsewhere. I find that method to be the most readable:
make/free foo = {1,2,3}
return foo
end
May 2, 2024 at 05:40 am - Permalink
tony's response is just about perfect. I will just add in my personal preference. I like to use multiple returns in order to use named variables in the function signature. I find that helps me remember what the return values represent.
-Kris
May 2, 2024 at 08:23 am - Permalink
Thank you for the suggestions. Tony's answer is a simply way to achieve what I need.
May 6, 2024 at 12:06 am - Permalink